Advertising SDK Standard iOS
iOS Ads SDK (from now on SDK) is the tool that helps monetize advertising inventory in your applications. SDK requests and display advertisement creatives in the host's app.
SDK provides the ability to download and display the following types of ad creatives in your application:
Banners:
HTML Banner (HTML advertising content) - HTML-markup banners are embedded inside the
CreativeView
hierarchy. For serving HTML, SDK usesWebKit > WKWebView
under the hood (built-in browser engine), which renders and displays HTML code.Image Banner - advertising content presented as links on pictures. SDK operates by these links on the pictures to render advertising content.
Media Banner (video, audio) - media advertising content based on VAST specifications.
For more information, see the section Display banner advertising.
Product:
- Product Creative is a particular type of advertising creative for RetailMedia platforms, using SKU product identifiers in the RetailMedia platform.
For more information, see the section Display product creative.
Before you start
You should know the following parameters required for initialization and use of SDK. Ask the SDK team to give them to you.
- bannerUrl - URL for banner creatives (HTML Banner, Image Banner, Media Banner). Eg:
"https://my.server.com/banner_creative"
. - productUrl - URL for product creative. Eg:
"https://my.server.com/product_creative"
.
SDK version:
Demo: Advertising SDK Example
Stable release: Advertising SDK Standard
Requirements
- Swift 5 and above
- iOS 12 and above
Installation
Install CocoaPods dependency.
To use iOS Ads SDK you need to install a dependency in your application’s Podfile
file:
pod 'SAAdvertisingSDKStandard'
or
pod 'SAAdvertisingSDKStandard', :git => 'https://github.com/solutionarchitectstech/mobile_sdk_release_ios.git', :tag => '{{ version }}'
SDK initialization
In order to initialize the SDK and create of ad placements by the developer should call TechAdvetrising.initialize()
method at the time of the application launch or at the moment of user authorization.
You must provide initConfig
or remoteConfigUrl
in the params, but if you submit both, then initConfig
will have higher priority.
// If you want to use internal 'CUSTOM' protocol in the SDK.
TechAdvertising.initialize(options: TechAdvertisingOptions(
sessionId: "YOUR_SESSION_ID",
storeUrl: "https://apps.apple.com/us/app/myapp/id12345678",
initConfig: .init(
core: .init(
bannerUrl: "https://YOUR_BANNER_CREATIVE_ENDPOINT",
productUrl: "https://YOUR_PRODUCT_CREATIVE_ENDPOINT"
)
),
debugMode: true,
httpHeaders: [
"Authorization": "Bearer CUSTOM_AUTH_TOKEN"
]
))
// Here is an example, if you want to use 'OpenRTB' protocol.
TechAdvertising.initialize(options: TechAdvertisingOptions(
sessionId: "YOUR_SESSION_ID",
storeUrl: "https://apps.apple.com/us/app/myapp/id12345678",
transactionProtocol: .OPEN_RTB(
publisherId: "YOUR_PUBLISHER_ID", // Optional, set this up here if you have it
appContentType: .OTHER, // Optional
appContentProductionQuality: .PROFESSIONALLY_PRODUCED, // Optional
appContentMediaRating: .ALL_AUDIENCES // Optional
),
initConfig: .init(
core: .init(
bannerUrl: "https://YOUR_OPENRTB_ENDPOINT"
)
),
debugMode: true,
httpHeaders: [
"Authorization": "Bearer CUSTOM_AUTH_TOKEN"
]
))
// If you already have your authorized user id here, then keep the following line enabled.
// Otherwise, remove it here and follow recommendations in the 'User (UID)' chapter below.
TechAdvertising.shared.uid = "YOUR_AUTHORIZED_USER_ID"
TechAdvertising.initialize(options: TechAdvertisingOptions)
public struct TechAdvertisingOptions {
public init(
sessionId: String,
storeUrl: String,
transactionProtocol: TransactionProtocol = .CUSTOM,
initConfig: InitConfig? = nil,
remoteConfigUrl: String? = nil,
debugMode: Bool = false,
httpHeaders: [String: String] = [:]
)
}
public class TechAdvertising {
public var uid: String?
}
sessionId
- Unique session id determining the current application session. There are types of mobile apps:- Online app (your application uses your business backend) - in this case, please provide the session id, which matches the server-side session of your backend - this is the preferable way.
- Offline app (your mobile doesn't use its business backend). If your app doesn't use the backend, then your mobile app can pass generated
UUID
value here. IMPORTANT: yourUUID
must be generated once on each first application startup. You must never re-generate it during the application lifecycle. Only if your app is terminated (removed from memory) and re-started again, only this triggers a newUUID
value.
storeUrl
- link to the app in the app store. Eg:https://apps.apple.com/us/app/myapp/id12345678
.transactionProtocol
- Ad transaction protocol (with specific options inside). This option determines the "Layer-3 Transaction" of AdCOM v1.0. SDK supports the following transaction protocols:CUSTOM
- Internal custom protocol (used by default).OPEN_RTB
- OpenRTB 2.6OPEN_RTB( publisherId: String? = nil, appContentType: RTBContext? = nil, appContentProductionQuality: RTBProductionQuality? = nil, appContentMediaRating: RTBMediaRating? = nil )
publisherId
- (optional) Unique identifier of publisher.appContentType
- (optional) Type of your application content (game, video, text, etc.). Refer to List: Content Contexts in AdCOM 1.0.appContentProductionQuality
- (optional) Production quality (spcific for your application content). Refer to List: Production Qualities in AdCOM 1.0.appContentMediaRating
- (optional) Media rating per IQG guidelines (specific for your application content). Refer to List: Media Ratings in AdCOM 1.0.
initConfig
- (optional) InitConfig object what might be used to setup endpoint URLs (in case if you don't useremoteConfigUrl
). If you use both InitConfig andremoteConfigUrl
parameters then InitConfig always have higher prio. This means the values from your InitConfig object override values retrieved fromremoteConfigUrl
endpoint.remoteConfigUrl
- (optional) Endpoint URL to retrieve InitConfig object from server. Useful to retrieve init parameters from server, instead to hardcode its in your app. An example of API response in JSON format:JSON{ "core": { "bannerUrl": "https://my.server.com/banner_creative", "productUrl": "https://my.server.com/product_creative" } }
debugMode
- a flag that enables debug messages to be written to the application log. Defaultfalse
.httpHeaders
- dictionary that forms specific HTTP headers. Default[:]
.TechAdvertising.shared.uid
- (optional) - see our recommendations (when to set / clean this value) in the section User ID (UID) below.
GEO location
SDK tries to detect current GEO location of user. We use it for better banner targeting. This means, you are (as a developer) have to add the following Privacy properties in the Info.plist
file of your application:
NSLocationWhenInUseUsageDescription="Here is you describe why your app uses GEO location permissions"
NSLocationUsageDescription="Here is you describe why your app uses GEO location permissions"
NSLocationAlwaysUsageDescription="Here is you describe why your app uses GEO location permissions"
NSLocationAlwaysAndWhenInUseUsageDescription="Here is you describe why your app uses GEO location permissions"
NSLocationTemporaryUsageDescriptionDictionary="Here is you describe why your app uses GEO location permissions"
Notice
If you skip these properties in your Info.plist
then LocationManager
will never return current GEO location. This means nil
value will always be used in each 'get advertisement' request. This means your app losses benefits of banner targeting features based on GEO location.
User ID (UID)
Your mobile application might be:
- Anonymous (public - no user based authorization at all). For example: any user can use whole functionality of your app without needs to register own user account. Public (anonymous) usage.
- AuthN/AuthZ based (user based authorization required). For example: Sign-in screen on startup.
- Mixed app (some screens of your app are publicly available for anyone, but other ones are only available for authorized users). For example: Anyone (without user account) can navigate between catalog / products screens. But if user tries to 'Add to cart' or 'To make an order', then application asks user to pass authorization (Sign-in / Sign-up).
IMPORTANT
Advertising SDK provides TechAdvertising.shared.uid
property. Please, setup this property with 'user id' value of your real authorized user.
You have to set this property on each successful 'Sign-in' attempt.
You can skip setup of this property only in one scenario: if your mobile is Anonymous and never uses user authorization.
Here is an example how to set TechAdvertising.shared.uid
property:
myAuthService.signIn(username, password) { authSession, error in
defer {
TechAdvertising.shared.uid = authSession.success ? authSession.user.id : nil
}
if let error = error {
print("ERROR: Unable to sign-in due error: \(error.localizedDescription)")
return
}
// Here, you do your own business logic specific for your mobile app
// after user passes authorization.
// Eg: navigate to 'Main' screen, or navigate to 'Cart' screen, etc ...
}
public class TechAdvertising {
public var uid: String?
}
uid
- (optional) Unique user ID, authorized in your mobile app.
Display banner
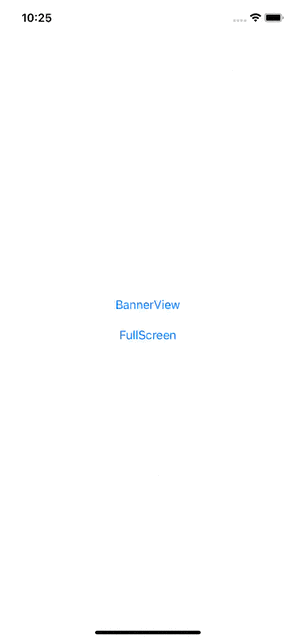
SDK provides the following types of banner creatives:
HTML Banner
This is an advertising creative that uses HTML content for advertising. SDK uses HTML rendering mechanisms to display this type of creatives (WebKit > WKWebView
). This implementation implies that an HTML page is added to the CreativeView
hierarchy, the advertising content. For example, as a banner, you can display a form questionnaire for users with controls, visual effects, and additional logic that can be performed using JavaScript.
Image Banner
This is an advertising creative that uses a picture file to display advertisements. It is an easy-to-use version of a banner for displaying advertising content in the form of a simple picture, which entails more incredible performance when displaying advertising and less delay on rendering content.
Media Banner (video, audio)
This creative is an advertising creative that uses VAST specifications to display media content as an advertisement.
To request and display ads, do the following steps:
- Add
CreativeView
to yourlayout
:
- Add `CreativeView` in the storyboard (xib) of your view.
- Connect your `creativeView` to necessary field of your view controller
using `@IBOutlet`
- Add `creative` property in your view controller, for example:
class MyViewController: UIViewController {
@IBOutlet weak var creativeView: CreativeView!
private var creative: Creative!
}
class MyViewController: UIViewController {
private var creative: Creative!
override func viewDidLoad() {
super.viewDidLoad()
// Let's create creative view and add it to your layout.
//
// You can also use CreativeView(frame: CGRect) constructor to instantiate the view
// with initial size/position.
// let creativeView = CreativeView(frame: CGRect(x: 0, y: 0, width: 640, height: 480))
let creativeView = CreativeView()
superview.addSubview(creativeView)
creativeView.translatesAutoresizingMaskIntoConstraints = false
// Here, you assign constraints to your creativeView.
. . .
// Optionally, you can disable adaptive UI using the following properties.
creativeView.isScrollEnabled = true // 'false' used by default
creativeView.scaleToFit = false // 'true' used by default
}
}
- Configure advertisement parameters for
CreativeView
using CreativeQuery:
class MyViewController: UIViewController {
@IBOutlet weak var creativeView: CreativeView!
private var creative: Creative!
override func viewDidLoad() {
super.viewDidLoad()
. . .
// If you use internal 'CUSTOM' protocol then use the following creative query
creativeView.query = CreativeQuery(
// NOTICE: Placement ID is required for 'CUSTOM' protocol
placementId: "YOUR_PLACEMENT_ID",
sizes: [SizeEntity(width: 260, height: 106)],
floorPrice: 2.0,
currency: "RUB",
customParams: [
"property1": "value1",
"property2": "value2"
]
)
// If 'OpenRTB' protocol then this example
creativeView.query = CreativeQuery(
// NOTICE: there is no `placementId` needed if 'OpenRTB' protocol is used.
sizes: [SizeEntity(width: 260, height: 106)],
// NOTICE: `markupType` is recommended if you use 'OpenRTB' protocol.
// Set this up as `.BANNER`, `.VIDEO`, `.AUDIO` or `.NATIVE` in your request query.
markupType: .BANNER,
floorPrice: 2.0,
currency: "RUB",
customParams: [
"property1": "value1",
"property2": "value2"
]
)
}
}
- Create Creative to control banner.
class MyViewController: UIViewController {
@IBOutlet weak var creativeView: CreativeView!
private var creative: Creative!
override func viewDidLoad() {
super.viewDidLoad()
. . .
// Let's init Creative
self.creative = .init(creativeViews: [creativeView])
self.creative.delegate = self
}
}
- Call
load
of Creative to load advertisement
class MyViewController: UIViewController {
@IBOutlet weak var creativeView: CreativeView!
private var creative: Creative!
override func viewDidLoad() {
super.viewDidLoad()
. . .
// Finally, let's load creatives
self.creative.load()
}
}
- You can customize error handling by using the protocol handler CreativeDelegate.
class MyViewController: UIViewController {
@IBOutlet weak var creativeView: CreativeView!
private var creative: Creative!
override func viewDidLoad() {
super.viewDidLoad()
. . .
// Here, we setup creative delegate
self.creative.delegate = self
}
}
extension MyViewController: CreativeDelegate {
public func onLoadDataSuccess(creativeView: CreativeView) {
let placementId = creativeView.query?.placementId
print("Creative.onLoadDataSuccess[\(String(describing: placementId))]")
}
public func onLoadDataFail(creativeView: CreativeView, error: Error) {
let placementId = creativeView.query?.placementId
print("Creative.onLoadDataFail[\(String(describing: placementId))]: \(error.localizedDescription)")
}
public func onLoadContentSuccess(creativeView: CreativeView) {
let placementId = creativeView.query?.placementId
print("Creative.onLoadContentSuccess[\(String(describing: placementId))]")
}
public func onLoadContentFail(creativeView: CreativeView, error: Error) {
let placementId = creativeView.query?.placementId
print("Creative.onLoadContentFail[\(String(describing: placementId))]: \(error.localizedDescription)")
}
public func onNoAdContent(creativeView: CreativeView) {
let placementId = creativeView.query?.placementId
print("Creative.onNoAdContent[\(String(describing: placementId))]")
}
public func onClose(creativeView: CreativeView) {
let placementId = creativeView.query?.placementId
print("Creative.onClose[\(String(describing: placementId))]")
}
}
Display dynamic collections
If you wish to use dynamic lists of banners, based on UICollectionViewController
, UICollectionViewCell
and dequeueReusableCell
, then please see our example code (use it as a template in your solution): SingleCollectionCreativeViewController.swift
This helps you:
- To avoid banner loading if user has not scrolled to necessary banner yet.
- To get smoothness UI effect of scrolling.
- To minimize memory-leak risks.
Display product creative
Product Banner - a special type of advertising creative for RetailMedia platforms, which uses the SKU product identifier in the RetailMedia platform. In this case, SDK does not render advertising content or perform tracking and landing page navigation functions. In the case of this advertising creative, it is the responsibility zone of the host developer. Having received a response from SDK with a list of creatives and their SKU, the developer forms a display of advertising content based on existing pictures of products in the platform on these SKUs, according to the internal templates and styles, providing a call for the necessary tracking of events.
class MyViewController: UIViewController {
private var productCreative: ProductCreative!
override func viewDidLoad() {
super.viewDidLoad()
. . .
// Let's setup product creative
self.productCreative = ProductCreative(query: ProductCreativeQuery(
placementId: "YOUR_PLACEMENT_ID",
customParams: [
"property1": "value1",
"property2": "value2"
]
))
self.productCreative.delegate = self
}
@IBAction func onRequestButtonClick(_ sender: Any) {
self.productCreative.load()
}
}
extension MyViewController: ProductCreativeDelegate {
public func onNoAdContent(query: ProductCreativeQuery) {
let placementId = query.placementId
print("Product.onNoAdContent[\(String(describing: placementId))]")
}
func onLoadDataSuccess() {
print("Product.onLoadDataSuccess")
}
func onLoadDataFail(error: Error) {
print("Product.onLoadDataFail: \(error.localizedDescription)")
}
func onLoadContentSuccess(entity: ProductCreativeEntity) {
print("Product.onLoadContentSuccess: \(String(describing: entity))")
}
func onLoadContentFail(query: ProductCreativeQuery, error: Error) {
print("Product.onLoadContentFail[\(query.placementId)]: \(error.localizedDescription)")
}
}
Helpers
InitConfig
SDK init config object. Сontains endpoint urls to request ad creatives.
public struct InitConfig {
public init(core: InitConfigCoreEntity? = nil)
}
core
- (optional) Core config of SDK. URls for common ad creatives (see InitConfigCoreEntity)
InitConfigCoreEntity
URl-config for common ad creatives
public struct InitConfigCoreEntity {
public init(
bannerUrl: String? = nil,
productUrl: String? = nil
)
}
bannerUrl
- (optional) URL for banner creative requestproductUrl
- (optional) URL for product creative request
CreativeView
View container which used to display retrieved advertisement inside (HTML Banner, Image Banner, Media Banner). All internal UI hierarchy is constructed automatically by our SDK based on obtained (from server) creative type.
public class CreativeView: UIView {
public var query: CreativeQuery?
public var scaleToFit: Bool = true
public var isScrollEnabled: Bool = false
public override init(frame: CGRect)
public required init?(coder: NSCoder)
}
isScrollEnabled
- enables or disables vertical and horizontal scrolling of HTML content. Defaultfalse
. inside ofCreativeView
frame. Eg: if you use complex HTML content as banner creative, but your app UI doesn't provide enough frame size to show whole HTML content. So, you can enable this option to give capability to your users to scroll the HTML content to see it.NOTICE
isScrollEnabled
property only affects to HTML based banners.scaleToFit
- this option fits embedded banner content to aspect ratio of yourCreativeView
frame. Defaulttrue
.query
- (optional) Banner creative query. Set this up before you start banner loading. If you keep this parameter asnil
then Creative controller ignores such banner view while banner loading. See more details in the CreativeQuery
Creative
Controller to load (to manage) banner creatives.
public class Creative {
public weak var delegate: CreativeDelegate?
public convenience init(creativeView: CreativeView)
public init(creativeViews: [CreativeView])
public func load(path: String = "")
}
init(creativeView: CreativeView)
- constructor, which manages single UI component:CreativeView
.init(creativeViews: [CreativeView])
- (multiple impressions) constructor, which manages collection of UI components:CreativeView
.creativeView
-CreativeView
UI container, used to display retrieved banner content.creativeViews
- list ofCreativeView
containers to load creatives with a single request using Multi Impression technology.
delegate
- (optional) CreativeDelegate callback delegate to handle loading process and lifecycle of banner(s).load()
- function to start loading process of banner(s), which were using in the controller init.path
- a path which will be concatinated to banner creative endpoint URL. Default""
.
FullscreenCreativeViewController
Controller to show banner creative in modal fullscreen mode.
class SingleFullscreenCreativeViewController: UIViewController {
private var fullscreenVC: FullscreenCreativeViewController!
override func viewDidLoad() {
let vc = FullscreenCreativeViewController()
vc.delegate = self
self.fullscreenVC = vc
}
@IBAction func onButtonClick(_ sender: Any) {
self.fullscreenVC.query = CreativeQuery(
placementId: "YOUR_PLACEMENT_ID",
sizes: [SizeEntity(width: 260, height: 106)],
customParams: [
"property1": "value1",
"property2": "value2"
]
)
self.present(fullscreenVC, animated: true)
}
}
// MARK: - CreativeDelegate
extension SingleFullscreenCreativeViewController: CreativeDelegate {
public func onLoadDataSuccess(creativeView: CreativeView) {
let placementId = creativeView.query?.placementId
print("onLoadDataSuccess[\(placementId ?? "")]")
}
public func onLoadDataFail(creativeView: CreativeView, error: Error) {
let placementId = creativeView.query?.placementId
print("onLoadDataFail[\(placementId ?? "")]: \(error.localizedDescription)")
}
public func onLoadContentSuccess(creativeView: CreativeView) {
let placementId = creativeView.query?.placementId
print("onLoadContentSuccess[\(placementId ?? "")]")
}
public func onLoadContentFail(creativeView: CreativeView, error: Error) {
let placementId = creativeView.query?.placementId
print("onLoadContentFail[\(placementId ?? "")]: \(error.localizedDescription)")
fullscreenVC.dismiss(animated: true)
}
public func onNoAdContent(creativeView: CreativeView) {
let placementId = creativeView.query?.placementId
print("onNoAdContent[\(placementId ?? "")]")
fullscreenVC.dismiss(animated: true)
}
public func onClose(creativeView: CreativeView) {
let placementId = creativeView.query?.placementId
print("onClose[\(placementId ?? "")]")
fullscreenVC.dismiss(animated: true)
}
}
public class FullscreenCreativeViewController: UIViewController {
public var query: CreativeQuery?
public var path = ""
public weak var delegate: CreativeDelegate?
public init()
public required init?(coder: NSCoder)
init()
,init?(coder: NSCoder)
- standard constructors inherited fromUIViewController
.query
- (optional) - Banner creative query. Set this up before you start banner loading. If you keep this parameter asnil
then Creative controller ignores such banner view while banner loading. See more details in the CreativeQuerydelegate
- (optional) CreativeDelegate callback delegate to handle loading process and lifecycle of banner(s).path
- a path which will be concatinated to banner creative endpoint URL. Default""
.
CreativeDelegate
Callback delegate to handle loading process and lifecycle of banner creative(s).
@objc public protocol CreativeDelegate: AnyObject {
@objc optional func onLoadDataSuccess(creativeView: CreativeView)
@objc optional func onLoadDataFail(creativeView: CreativeView, error: Error)
@objc optional func onLoadContentSuccess(creativeView: CreativeView)
@objc optional func onLoadContentFail(creativeView: CreativeView, error: Error)
@objc optional func onNoAdContent(creativeView: CreativeView)
@objc optional func onClose(creativeView: CreativeView)
}
onLoadDataSuccess
- (optional) a request to the ad server returned creative data, content loading is started;onLoadDataFail
- (optional) an error when requesting the ad server;onLoadContentSuccess
- (optional) the creative was successfully loaded;onLoadContentFail
- (optional) error while loading the creatives;onNoAdContent
- (optional) server returned 204 HTTP code;onClose
- (optional) banner has been closed (eg: user pressed the close button of the banner);
CreativeQuery
Query criteria to request banner creative.
public struct CreativeQuery {
public var placementId: String
public var sizes: [SizeEntity]
public var markupType: MarkupType?
public var floorPrice: Double?
public var currency: String?
public var customParams: [String: String]
public init(
placementId: String = "",
sizes: [SizeEntity],
markupType: MarkupType? = nil
floorPrice: Double? = nil,
currency: String? = nil,
customParams: [String: String] = [:]
)
}
placementId
- ID of the ad slot. Set this up in your query if you use 'CUSTOM' protocol in the SDK initialization. Eg:123456
. Skip it (don't set it) if you use 'OpenRTB' protocol.sizes
- list of valid sizes for the banner. Default[]
. Eg:[SizeEntity(width: 260, height: 106)]
.markupType
- (optional) Type of the creative markup (.BANNER
,.VIDEO
,.AUDIO
,.NATIVE
). This property is recommended if you use 'OpenRTB' protocol. Here you specify what kind of creative you expect to receive from a server.floorPrice
- (optional) minimal show price.currency
- (optional) currency of payment.customParams
- custom parameters to add to banner request. Default[:]
. Eg:["example": "value", "example2": "value2"]
. NOTICE: JSON-compatible object must be passed only, otherwise runtime error (onLoadDataFail()
). Eg:SwiftcustomParams: [ "sku": [ "id": "LG00001", "name": "Lego bricks (speed boat)", "extra": [ "variances": ["Medium", "Electrical"], "points": 33.20 ] ], "categories": ["Kids", "SpecialOffer", "2025"], "gdprConsent": "CPsmEWIPsmEWIABAMBFRACBsABEAAAAgEIYgACJAAYiAAA.QRXwAgAAgivA", "ccpa": "1YNN", "coppa": 1, "discount": 0.20, "contacts": [ [ "name" to "John Doe", "email" to "john.doe@mail.me" ], [ "name" to "Jane Groovy", "email" to "jane.groovy@mail.me" ], ] ]
Size
SizeEntity
The object describing the size of an ad placement. When initialized takes two integers corresponding to the width and height of an ad placemnt in pixels.
SizeEntity(width: 300, height: 250)
public struct SizeEntity {
public let width: Int
public let height: Int
}
width
- Width in pixels. Eg:300
height
- Height in pixels. Eg:250
ProductCreative
Controller to load (to manage) product creatives.
public class ProductCreative {
public weak var delegate: ProductCreativeDelegate?
public convenience init(query: ProductCreativeQuery)
public init(queries: [ProductCreativeQuery])
public func load(path: String = "")
}
init(query: ProductCreativeQuery)
- constructor which used to manage singe product creative. See ProductCreativeQuery.init(queries: [ProductCreativeQuery])
- (multiple impressions) constructor, which manages collection of product creatives. See ProductCreativeQuery.delegate
- (optional) ProductCreativeDelegate callback delegate to handle loading process and lifecycle of product(s).load()
- function to start loading process of product(s), which queries were using in the controller init.path
- a path which will be concatinated to product creative endpoint URL. Default""
.
ProductCreativeQuery
Query criteria of requested product creative.
public struct ProductCreativeQuery {
public var placementId: String
public var customParams: [String: String]
public init(placementId: String, customParams: [String: String] = [:]) {
self.placementId = placementId
self.customParams = customParams
}
}
placementId
- ID of the ad slot.customParams
- custom parameters what might be added to request to retrieve ad creative. Default[:]
. NOTICE: JSON-compatible object must be passed only, otherwise runtime error (onLoadDataFail()
). Eg:SwiftcustomParams: [ "sku": [ "id": "LG00001", "name": "Lego bricks (speed boat)", "extra": [ "variances": ["Medium", "Electrical"], "points": 33.20 ] ], "categories": ["Kids", "SpecialOffer", "2025"], "gdprConsent": "CPsmEWIPsmEWIABAMBFRACBsABEAAAAgEIYgACJAAYiAAA.QRXwAgAAgivA", "ccpa": "1YNN", "coppa": 1, "discount": 0.20, "contacts": [ [ "name" to "John Doe", "email" to "john.doe@mail.me" ], [ "name" to "Jane Groovy", "email" to "jane.groovy@mail.me" ], ] ]
ProductCreativeDelegate (#product_creative_delegate)
public protocol ProductCreativeDelegate: AnyObject {
func onLoadDataSuccess()
func onLoadDataFail(error: Error)
func onLoadContentSuccess(entity: ProductCreativeEntity)
func onLoadContentFail(query: ProductCreativeQuery, error: Error)
func onNoAdContent(query: ProductCreativeQuery)
}
onLoadDataSuccess
- product creative request success, content loading is started;onLoadDataFail
- product creative request fails due an error;onLoadContentSuccess
- loading of product creative content success; See ProductCreativeEntity;onLoadContentFail
- loading of product creative content fails due an error; See ProductCreativeQuery;onNoAdContent
- server returned 204 HTTP code;
ProductCreativeEntity
public struct ProductCreativeEntity {
public let requestId: String
public let placementId: String
public var sku: String
public var disclaimer: String?
public var externalId: String
public var tracking: ProductCreativeTrackingEntity?
public var advertiser: String?
}
requestId
- id of requestplacementId
- unique placement identifiersku
- unique product identifierdisclaimer
- (optional) written disclaimer or disclaimer of liability when publishing advertising contentexternalId
- external unique ad identifiertracking
- (optional) object containing URLs for event tracking ProductCreativeTrackingEntityadvertiser
- (optional) advertiser that provide ad source
ProductCreativeTrackingEntity
Object represents tracking urls of requested product creative. Which must be invoked on your mobile app side.
public struct ProductCreativeTrackingEntity {
public var impression: [String] = []
public var click: [String] = []
public var view: [String] = []
}
click
- collection of urls to track click on ads. Default[]
.impression
- collection of urls to track impression of ads. Default[]
.view
- list of urls to track viewability of ads. Default[]
.